Active Admin and Paperclip done right
Active Admin is an administration framework for Ruby on Rails applications which helps in creating beautiful administration panels. Paperclip is a file attachment library for Active Record which makes management of files to models really easy.
Recently while creating administration page for a model, in which Paperclip was being used for managing attachments, I oberved that Active Admin was not handling it properly. For example, the view page was showing as below:
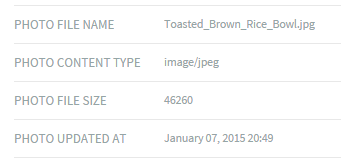
And the edit and new page was being shown as below:
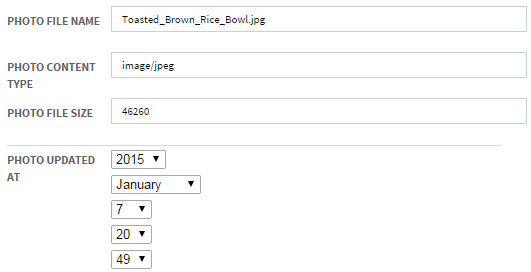
There was no way one could upload the images with above interface. Hence I scoured internet and found some nifty hacks. Let’s go through each of them:
View Page
Instead of showing attached image properties, I wanted to show the image itself. Also for other attached files types (like pdf and doc), I wanted to give an URL. Below code achieved the same:
1
2
3
4
5
6
7
8
9
10
11
12
13
ActiveAdmin.register Item do
show do |recipe|
attributes_table do
row :instruction do
item.instruction? ? link_to(item.instruction_file_name, item.instruction.url) : content_tag(:span, "No instruction file yet")
end
row :photo do
item.photo? ? image_tag(item.photo.url, height: '100') : content_tag(:span, "No photo yet")
end
end
active_admin_comments
end
end
This resulted in following:
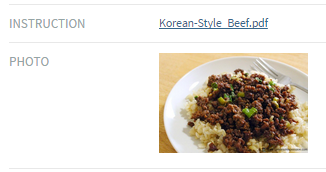
New and Edit Page
I wanted to show an upload button to upload files. Also I wanted to show current file attached on Edit page so users can verify the attached content before modifying it. The code below achieved the same:
1
2
3
4
5
6
7
8
9
ActiveAdmin.register Item do
form :html => { :enctype => "multipart/form-data" } do |f|
f.inputs do
f.input :instruction, hint: f.item.instruction? ? link_to(f.item.instruction_file_name, f.item.instruction.url) : content_tag(:span, "Upload PDF file")
f.input :photo, hint: f.item.photo? ? image_tag(f.item.photo.url, height: '100') : content_tag(:span, "Upload JPG/PNG/GIF image")
end
f.actions
end
end
This resulted in following:
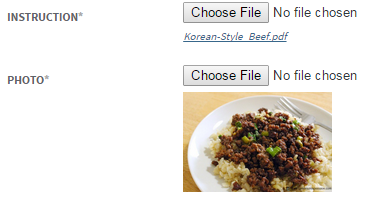
Note that you have to set enctype
attribute in your form to multipart/form-data
to enable upload of files.
And that’s it. I was pretty happy with the end result. Do let me know in comments if you happen to know any other trick.